Welcome to my CORS training site!
Here, you can easily test different CORS configurations. Some requests are made to the same origin, while others are sent to a remote API.
You can find my GitHub repository by clicking here
Tutorial
Inspecting Your Developer Tools
To inspect your developer tools, right-click and select "Inspect". Next, go to the "Network" tab and make sure all requests are displayed.
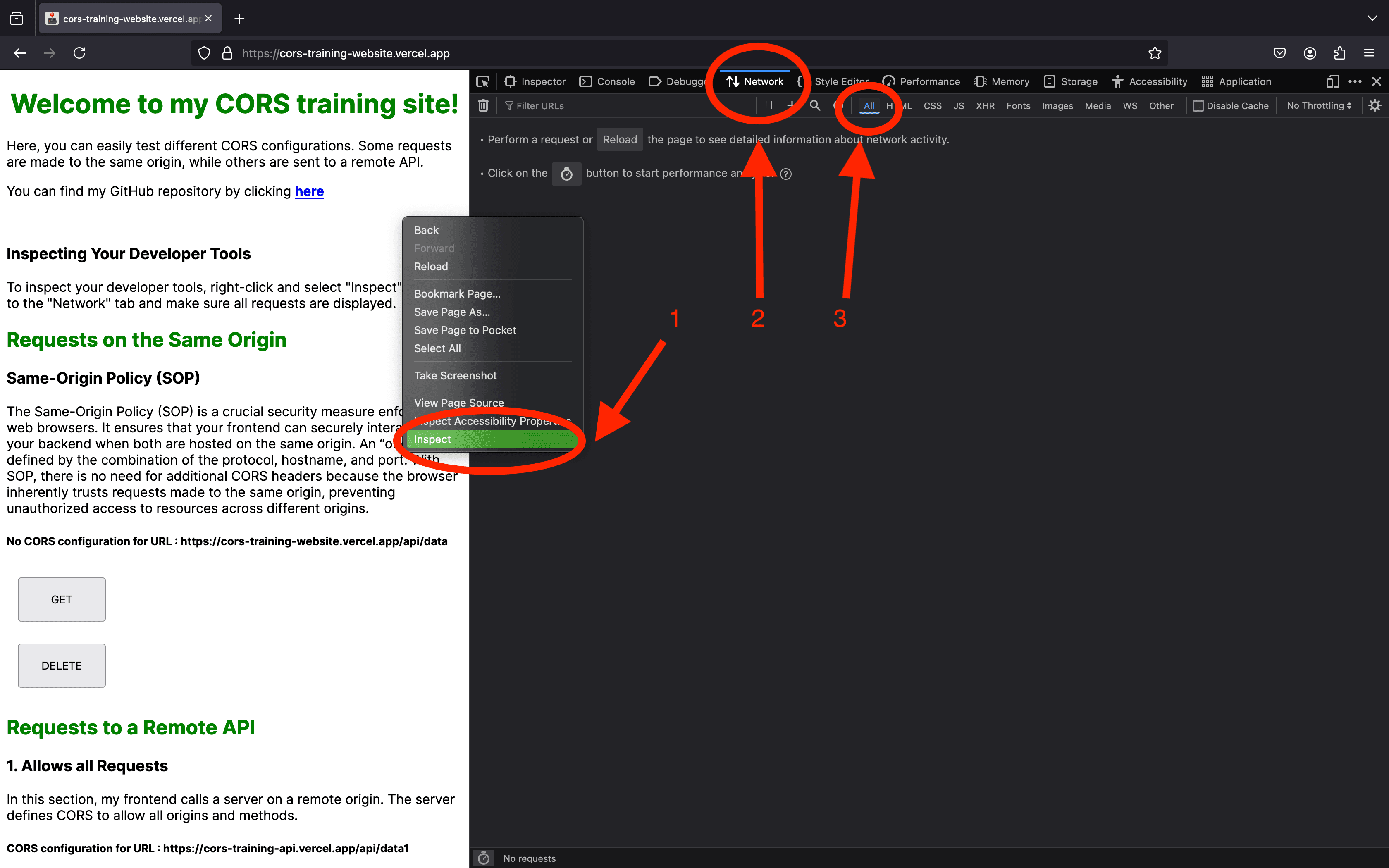
Then, let's investigate
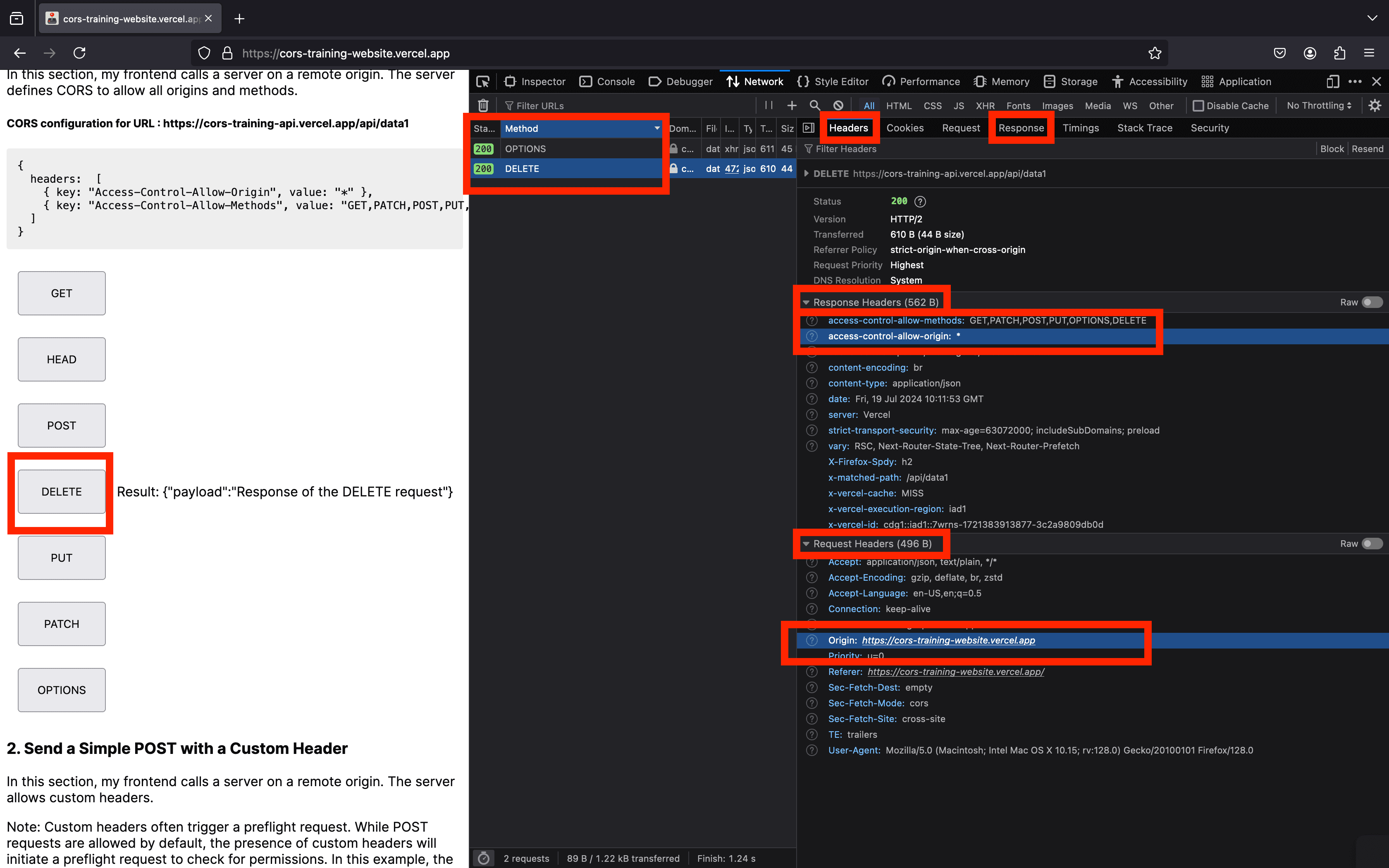
Requests on the Same Origin
Same-Origin Policy (SOP)
The Same-Origin Policy (SOP) is a crucial security measure enforced by web browsers. It ensures that your frontend can securely interact with your backend when both are hosted on the same origin. An “origin“ is defined by the combination of the protocol, hostname, and port. With SOP, there is no need for additional CORS headers because the browser inherently trusts requests made to the same origin, preventing unauthorized access to resources across different origins.
No CORS configuration for URL : https://cors-training-website.vercel.app/api/data
Requests to a Remote API
1. Allows all Requests
In this section, my frontend calls a server on a remote origin. The server defines CORS to allow all origins and methods.
CORS configuration for URL : https://cors-training-api.vercel.app/api/data1
{
headers: [
{ key: "Access-Control-Allow-Origin", value: "*" },
{ key: "Access-Control-Allow-Methods", value: "GET,PATCH,POST,PUT,OPTIONS,DELETE" }
]
}
2. Send a Simple POST with a Custom Header
In this section, my frontend calls a server on a remote origin. The server allows custom headers.
Note: Custom headers often trigger a preflight request. While POST requests are allowed by default, the presence of custom headers will initiate a preflight request to check for permissions. In this example, the custom header is allowed.
CORS configuration for URL : https://cors-training-api.vercel.app/api/data2
{
headers: [
{ key: "Access-Control-Allow-Origin", value: "*" },
{ key: "Access-Control-Allow-Methods", value: "" },
{ key: "Access-Control-Allow-Headers", value: "Content-Type, Authorization, X-Custom-Header" }
]
}
3. Allow Only My Origin
In this section, my frontend calls a server on a remote origin. The server defines CORS to accept only my origin.
CORS configuration for URL : https://cors-training-api.vercel.app/api/data3
{
headers: [
{ key: "Access-Control-Allow-Origin", value: "https://cors-training-website.vercel.app" },
{ key: "Access-Control-Allow-Methods", value: "DELETE" }
]
}
4. Allow Only the PUT Method
In this section, my frontend calls a server on a remote origin. The server defines CORS to accept any website but only the "PUT" method.
CORS configuration for URL : https://cors-training-api.vercel.app/api/data4
{
headers: [
{ key: "Access-Control-Allow-Origin", value: "*" },
{ key: "Access-Control-Allow-Methods", value: "PUT" }
]
}
5. Allow Only "https://other-website.com"
In this section, my frontend calls a server on a remote origin. The server defines CORS to accept only "https://other-website.com" without any specific methods.
Note: Notice how the DELETE request is not even sent because it is not allowed by the server‘s CORS policy.
CORS configuration for URL : https://cors-training-api.vercel.app/api/data5
{
headers: [
{ key: "Access-Control-Allow-Origin", value: "https://other-website.com" }
]
}
6. Allow My Origin but with the Wrong Protocol
In this section, my frontend calls a server on a remote origin. The server defines CORS to accept my domain and port but not my protocol.
CORS configuration for URL : https://cors-training-api.vercel.app/api/data6
{
headers: [
{ key: "Access-Control-Allow-Origin", value: "http://cors-training-website.vercel.app" },
{ key: "Access-Control-Allow-Methods", value: "DELETE" }
]
}
7. Allow My Origin but with the Wrong Port
In this section, my frontend calls a server on a remote origin. The server defines CORS to accept my domain and protocol but not my port.
CORS configuration for URL : https://cors-training-api.vercel.app/api/data7
{
headers: [
{ key: "Access-Control-Allow-Origin", value: "http://cors-training-website.vercel.app:7404" },
{ key: "Access-Control-Allow-Methods", value: "DELETE" }
]
}
8. Allow a Higher Domain
In this section, my frontend calls a server on a remote origin. The server defines CORS to accept a higher domain than mine.
CORS configuration for URL : https://cors-training-api.vercel.app/api/data8
{
headers: [
{ key: "Access-Control-Allow-Origin", value: "https://vercel.app" },
{ key: "Access-Control-Allow-Methods", value: "DELETE" }
]
}
9. Explicit Default Port
In this section, my frontend calls a server on a remote origin while explicitly setting the default port.
CORS configuration for URL : https://cors-training-api.vercel.app/api/data9
{
headers: [
{ key: "Access-Control-Allow-Origin", value: "https://cors-training-website.vercel.app:443" },
{ key: "Access-Control-Allow-Methods", value: "DELETE" }
]
}